Your 1st AsyncAPI on Kafka mock
🗓️ Last updated on May 13, 2024 | 1 | Improve this page🪄 To Be Created
This is a new tutorial page that has to be written as part of our Refactoring Effort.
Goal of this page
- Write an AsyncAPI specification with Microcks convention
- Import it into Microcks
- Play with exposed mock endpoints
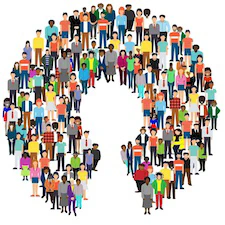
Still Didn’t Find Your Answer?
Join our community and get the help you need. Engage with other members, ask questions, and share knowledge to resolve your queries and expand your understanding.
Join the community